In this post, we will be integrating SonarQube with JaCoCo, the code coverage tool.
SonarQube Overview
SonarQube is a open source tool that aims to improve the quality of your code by measuring different metrics like code coverage, bugs, code smells and security vulnerabilities. It is a standalone service that can be deployed somewhere else and can be integrated within your code. SonarQube typically scans the entire code you publish to its server and generates a report based on it. This report is used to improvise and track your code.
I believe you already have a good understanding of SonarQube installation process and if not you hop on to the following link and get back to this post.
JaCoCo Overview
SonarQube also provide good understanding of code that has been exercised for testing generating code coverage report. Code coverage is a measure of how much code has been exercised for testing through development. It is one of the key metrics that one’s should follow in development as it will relatively make the application bug free.
We will be using JaCoCo for generating code coverage report. Jacoco is by far the most actively code coverage tool for Java ecosystem. Jacoco helps in estimating the code coverage for hundreds of lines of code written. It is a metric to measure the lines of code tested during development which assures the code has relatively less bug.
Add and Configure JaCoCo Plugin in Maven
Now, let’s add the dependency:
<plugin>
<groupId>org.jacoco</groupId>
<artifactId>jacoco-maven-plugin</artifactId>
<version>0.8.6</version>
<executions>
<execution>
<goals>
<goal>prepare-agent</goal>
</goals>
</execution>
<execution>
<id>report</id>
<phase>prepare-package</phase>
<goals>
<goal>report</goal>
</goals>
</execution>
</executions>
</plugin>
Code Coverage Report
After updating pom.xml, run the test or simply run command mvn clean install. It will create a coverage report at target/site/jacoco/index.html page.
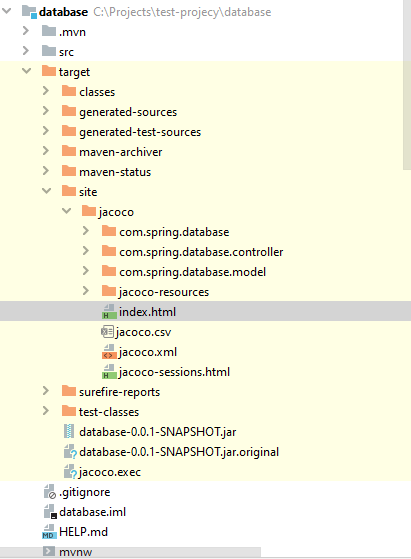
Once you open the index.html in browser, you will see something like this.
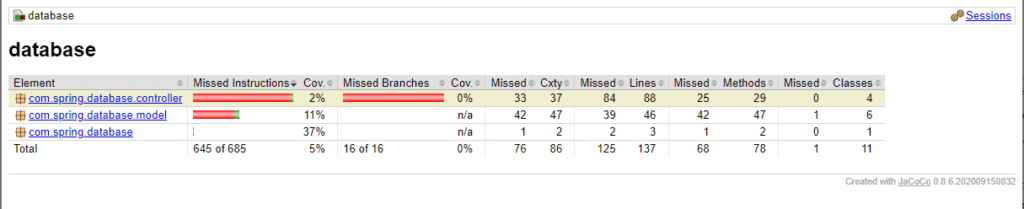
You can investigate each file and see the test coverage of it. Red means the code has not been exercised for testing, green means the code has been exercised during testing or code has been tested. This is the Red you need to exercise for testing and improve the coverage. Green means you did a good job of adding test cases to the given lines of code or methods. Let’s look into my controller.

All of them are Red as i have not added any test to it.
Set Code Coverage Limit for Maven Project
There are different ways to configure code coverage with Jacoco. Let’s update pom file to enforce the code coverage limit. Here’s an example:
<plugin>
<groupId>org.jacoco</groupId>
<artifactId>jacoco-maven-plugin</artifactId>
<version>0.8.6</version>
<executions>
<execution>
<goals>
<goal>prepare-agent</goal>
</goals>
</execution>
<execution>
<id>report</id>
<phase>prepare-package</phase>
<goals>
<goal>report</goal>
</goals>
</execution>
</executions>
<execution>
<goals>
<goal>check</goal>
</goals>
<configuration>
<rules>
<rule>
<element>PACKAGE</element>
<limits>
<limit>
<counter>LINE</counter>
<value>COVEREDRATIO</value>
<minimum>0.50</minimum>
</limit>
</limits>
</rule>
</rules>
</configuration>
</execution>
</plugin>
CoveredRatio 0.5 means 50%; the value should be between 0 to 1. 1 means 100%. Instead of enforcing line coverage, we can also facilitates other entities like BRANCH, CLASS, METHOD and INSTRUCTION. Along with it, we can also measure other metrics like COVEREDCOUNT, MISSEDCOUNT, MISSEDRATIO and TOTALCOUNT.
If the limit is not respected, it should throw error something like this while trying to build the project.

Integrate SonarQube with JaCoCo
The integration is straight forward. SonarQube should be running on the server; in enterprise SonarQube is running somewhere on a server instead locally. However, we will be running SonarQube locally. Run the command once plugin for JaCoCo is added.
mvn sonar:sonar -Dsonar.host.url=http://localhost:9000 -Dsonar.login=the-generated-token
Well, the code coverge report is sent to SonarQube Dashbord and should look something like this.

Conclusion
In this post, we learned how to integrate JaCoCo with SonarQube for better development approach. JaCoCo plugin generates code coverage reports for Java projects which can be used to practice better development approach with effective testing.
JaCoCo will execute the log of code coverage and let the developer know if the rules are respected or not. If not then developer will continue to add test code until the limit is respected.